2021-12-17 07:53:31 +01:00
# Hono
2021-12-14 21:17:56 +01:00
2022-02-07 07:44:33 +01:00
Hono[炎] - _**means flame🔥 in Japanese**_ - is small, simple, and ultrafast web framework for a Service Workers API based serverless such as Cloudflare Workers and Fastly Compute@Edge.
2021-12-17 07:53:31 +01:00
```js
2022-01-09 13:10:12 +01:00
import { Hono } from 'hono'
2022-01-01 07:29:41 +01:00
const app = new Hono()
2021-12-17 07:53:31 +01:00
2022-01-05 21:11:37 +01:00
app.get('/', (c) => c.text('Hono!!'))
2021-12-17 07:53:31 +01:00
app.fire()
```
2022-01-08 01:48:22 +01:00
## Features
2021-12-17 07:53:31 +01:00
2022-02-09 15:14:44 +01:00
- **Ultra fast** - the router is implemented with Trie-Tree structure. Not use loops.
2022-01-12 01:20:16 +01:00
- **Zero dependencies** - using only Web standard API.
- **Middleware** - builtin middleware, and you can make your own middleware.
- **Optimized** - for Cloudflare Workers.
2021-12-14 21:17:56 +01:00
2021-12-27 17:34:09 +01:00
## Benchmark
2022-01-05 21:11:37 +01:00
**Hono is fastest** compared to other routers for Cloudflare Workers.
2022-01-05 10:41:29 +01:00
2022-01-08 21:30:50 +01:00
```plain
2022-01-26 14:11:11 +01:00
hono x 708,671 ops/sec ±2.58% (58 runs sampled)
itty-router x 159,610 ops/sec ±2.86% (87 runs sampled)
sunder x 322,846 ops/sec ±2.24% (86 runs sampled)
worktop x 223,625 ops/sec ±2.01% (95 runs sampled)
2021-12-27 17:34:09 +01:00
Fastest is hono
2022-01-26 14:11:11 +01:00
✨ Done in 57.83s.
2021-12-27 17:34:09 +01:00
```
2022-01-05 21:11:37 +01:00
## Hono in 1 minute
2022-01-08 01:48:22 +01:00
Below is a demonstration to create an application of Cloudflare Workers with Hono.
2022-01-05 21:11:37 +01:00
2022-02-01 14:15:00 +01:00
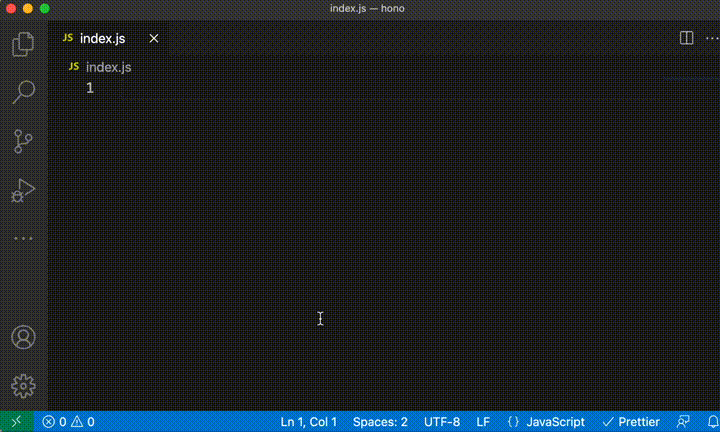
2022-01-05 21:11:37 +01:00
2021-12-14 21:17:56 +01:00
## Install
2022-01-08 01:48:22 +01:00
You can install from npm registry:
2022-01-05 21:11:37 +01:00
2022-01-08 21:30:50 +01:00
```sh
yarn add hono
2021-12-14 21:17:56 +01:00
```
or
```sh
2022-01-08 21:30:50 +01:00
npm install hono
2021-12-14 21:17:56 +01:00
```
2021-12-20 03:58:40 +01:00
## Methods
2022-01-05 21:11:37 +01:00
Instance of `Hono` has these methods:
2022-01-05 10:41:29 +01:00
- app.**HTTP_METHOD**(path, handler)
- app.**all**(path, handler)
2021-12-20 03:58:40 +01:00
- app.**route**(path)
2021-12-20 23:34:38 +01:00
- app.**use**(path, middleware)
2022-01-05 21:19:16 +01:00
- app.**fire**()
2022-01-12 01:20:16 +01:00
- app.**fetch**(request, env, event)
2021-12-20 03:58:40 +01:00
2021-12-17 07:53:31 +01:00
## Routing
### Basic
2021-12-14 21:17:56 +01:00
2021-12-20 03:58:40 +01:00
`app.HTTP_METHOD`
2021-12-14 21:17:56 +01:00
```js
2021-12-20 23:34:38 +01:00
// HTTP Methods
2022-01-12 18:29:11 +01:00
app.get('/', (c) => c.text('GET /'))
app.post('/', (c) => c.text('POST /'))
2021-12-20 23:34:38 +01:00
// Wildcard
2022-01-12 18:29:11 +01:00
app.get('/wild/*/card', (c) => {
return c.text('GET /wild/*/card')
2021-12-20 23:34:38 +01:00
})
2021-12-17 07:53:31 +01:00
```
2021-12-14 21:17:56 +01:00
2021-12-20 03:58:40 +01:00
`app.all`
```js
2021-12-20 23:34:38 +01:00
// Any HTTP methods
2022-01-12 18:29:11 +01:00
app.all('/hello', (c) => c.text('Any Method /hello'))
2021-12-20 03:58:40 +01:00
```
2021-12-17 07:53:31 +01:00
### Named Parameter
2021-12-14 21:17:56 +01:00
2021-12-17 07:53:31 +01:00
```js
2021-12-21 09:37:02 +01:00
app.get('/user/:name', (c) => {
2022-01-26 04:38:20 +01:00
const name = c.req.param('name')
2021-12-17 07:53:31 +01:00
...
})
```
### Regexp
```js
2021-12-21 09:37:02 +01:00
app.get('/post/:date{[0-9]+}/:title{[a-z]+}', (c) => {
2022-01-26 04:38:20 +01:00
const date = c.req.param('date')
const title = c.req.param('title')
2021-12-17 07:53:31 +01:00
...
2021-12-14 21:17:56 +01:00
```
2021-12-17 07:53:31 +01:00
### Chained Route
```js
app
.route('/api/book')
2021-12-21 09:37:02 +01:00
.get(() => {...})
.post(() => {...})
.put(() => {...})
2021-12-17 07:53:31 +01:00
```
2021-12-14 20:26:22 +01:00
2022-01-27 01:09:54 +01:00
### Custom 404 Response
You can customize 404 Not Found response:
```js
app.get('*', (c) => {
return c.text('Custom 404 Error', 404)
})
```
2022-02-15 08:52:06 +01:00
### no strict
If `strict` is set `false` , `/hello` and`/hello/` are treated the same:
```js
const app = new Hono({ strict: false })
app.get('/hello', (c) => c.text('/hello or /hello/'))
```
2022-01-05 21:11:37 +01:00
## async/await
2022-01-03 10:11:46 +01:00
```js
2022-01-12 18:29:11 +01:00
app.get('/fetch-url', async (c) => {
2022-01-03 10:11:46 +01:00
const response = await fetch('https://example.com/')
2022-01-12 18:29:11 +01:00
return c.text(`Status is ${response.status}`)
2022-01-03 10:11:46 +01:00
})
```
2021-12-20 03:58:40 +01:00
## Middleware
2022-01-01 08:22:35 +01:00
### Builtin Middleware
```js
2022-02-01 14:15:00 +01:00
import { Hono } from 'hono'
import { poweredBy } from 'hono/powered-by'
import { logger } from 'hono/logger'
import { basicAuth } from 'hono/basicAuth'
2022-01-01 08:22:35 +01:00
2022-02-01 14:15:00 +01:00
const app = new Hono()
2022-01-01 08:22:35 +01:00
2022-02-01 14:15:00 +01:00
app.use('*', poweredBy())
app.use('*', logger())
2022-01-08 01:48:22 +01:00
app.use(
'/auth/*',
2022-02-01 14:15:00 +01:00
basicAuth({
2022-01-08 01:48:22 +01:00
username: 'hono',
password: 'acoolproject',
})
)
2022-02-01 14:15:00 +01:00
...
2022-01-01 08:22:35 +01:00
```
2022-01-08 01:48:22 +01:00
Available builtin middleware are listed on [src/middleware ](https://github.com/yusukebe/hono/tree/master/src/middleware ).
2022-01-05 21:11:37 +01:00
2022-01-01 08:22:35 +01:00
### Custom Middleware
2022-01-05 21:11:37 +01:00
You can write your own middleware:
2021-12-20 03:58:40 +01:00
```js
2022-01-05 10:41:29 +01:00
// Custom logger
app.use('*', async (c, next) => {
2021-12-21 09:37:02 +01:00
console.log(`[${c.req.method}] ${c.req.url}`)
2022-01-03 10:11:46 +01:00
await next()
2022-01-05 10:41:29 +01:00
})
2021-12-20 03:58:40 +01:00
2022-01-08 01:48:22 +01:00
// Add a custom header
2022-01-05 10:41:29 +01:00
app.use('/message/*', async (c, next) => {
2022-01-03 10:11:46 +01:00
await next()
2022-02-09 15:14:44 +01:00
await c.header('x-message', 'This is middleware!')
2022-01-05 10:41:29 +01:00
})
2021-12-20 18:35:03 +01:00
2022-01-12 18:29:11 +01:00
app.get('/message/hello', (c) => c.text('Hello Middleware!'))
2021-12-20 03:58:40 +01:00
```
2022-01-16 13:34:06 +01:00
### Handling Error
```js
app.use('*', async (c, next) => {
try {
await next()
} catch (err) {
console.error(`${err}`)
2022-01-27 01:09:54 +01:00
c.res = c.text('Custom Error Message', { status: 500 })
2022-01-16 13:34:06 +01:00
}
})
```
2021-12-21 09:37:02 +01:00
## Context
2022-02-09 15:14:44 +01:00
To handle Request and Reponse, you can use Context object:
2022-01-05 21:11:37 +01:00
### c.req
2021-12-21 09:37:02 +01:00
```js
2022-01-05 10:41:29 +01:00
// Get Request object
2021-12-21 09:37:02 +01:00
app.get('/hello', (c) => {
2022-01-01 16:32:01 +01:00
const userAgent = c.req.headers.get('User-Agent')
2021-12-21 09:37:02 +01:00
...
})
2022-01-05 10:41:29 +01:00
2022-01-26 04:38:20 +01:00
// Shortcut to get a header value
app.get('/shortcut', (c) => {
const userAgent = c.req.header('User-Agent')
...
})
2022-01-05 10:41:29 +01:00
// Query params
app.get('/search', (c) => {
const query = c.req.query('q')
...
})
// Captured params
app.get('/entry/:id', (c) => {
2022-01-26 04:38:20 +01:00
const id = c.req.param('id')
2022-01-05 10:41:29 +01:00
...
})
2021-12-21 09:37:02 +01:00
```
2022-01-26 04:38:20 +01:00
### Shortcuts for Response
2022-01-12 01:20:16 +01:00
```js
2022-01-26 04:38:20 +01:00
app.get('/welcome', (c) => {
c.header('X-Message', 'Hello!')
c.header('Content-Type', 'text/plain')
c.status(201)
2022-01-29 01:09:37 +01:00
2022-01-26 04:38:20 +01:00
return c.body('Thank you for comming')
2022-01-29 01:09:37 +01:00
2022-01-26 04:38:20 +01:00
/*
Same as:
return new Response('Thank you for comming', {
status: 201,
2022-02-05 14:20:34 +01:00
statusText: 'Created',
2022-01-26 04:38:20 +01:00
headers: {
'X-Message': 'Hello',
2022-01-27 01:09:54 +01:00
'Content-Type': 'text/plain',
'Content-Length: '22'
2022-01-26 04:38:20 +01:00
}
})
*/
2022-01-12 01:20:16 +01:00
})
```
2022-01-05 21:11:37 +01:00
### c.text()
Render text as `Content-Type:text/plain` :
2021-12-20 03:58:40 +01:00
```js
2022-01-05 10:41:29 +01:00
app.get('/say', (c) => {
return c.text('Hello!')
2021-12-20 03:58:40 +01:00
})
```
2022-01-05 21:11:37 +01:00
### c.json()
2022-01-08 05:51:47 +01:00
Render JSON as `Content-Type:application/json` :
2022-01-05 19:22:53 +01:00
```js
app.get('/api', (c) => {
return c.json({ message: 'Hello!' })
})
```
2022-01-08 05:51:47 +01:00
### c.html()
Render HTML as `Content-Type:text/html` :
```js
2022-01-09 13:46:46 +01:00
app.get('/', (c) => {
2022-01-08 05:51:47 +01:00
return c.html('< h1 > Hello! Hono!< / h1 > ')
})
```
2022-01-09 13:46:46 +01:00
### c.redirect()
Redirect, default status code is `302` :
```js
app.get('/redirect', (c) => c.redirect('/'))
app.get('/redirect-permanently', (c) => c.redirect('/', 301))
```
2022-01-26 04:38:20 +01:00
### c.res
```js
// Response object
app.use('/', (c, next) => {
next()
c.res.headers.append('X-Debug', 'Debug message')
})
```
### c.event
```js
// FetchEvent object
app.use('*', async (c, next) => {
c.event.waitUntil(
...
)
await next()
})
```
### c.env
```js
// Environment object for Cloudflare Workers
app.get('*', async c => {
const counter = c.env.COUNTER
...
})
```
2022-01-05 21:19:16 +01:00
## fire
`app.fire()` do:
```js
addEventListener('fetch', (event) => {
event.respondWith(this.handleEvent(event))
})
```
2022-01-12 01:20:16 +01:00
## fetch
`app.fetch()` is for Cloudflare Module Worker syntax.
```js
export default {
fetch(request: Request, env: Env, event: FetchEvent) {
return app.fetch(request, env, event)
},
}
2022-01-30 03:33:17 +01:00
/*
or just do this:
export default app
*/
2022-01-12 01:20:16 +01:00
```
2022-01-05 21:11:37 +01:00
## Cloudflare Workers with Hono
2022-01-02 14:46:36 +01:00
2022-01-07 09:34:12 +01:00
Using `wrangler` or `miniflare` , you can develop the application locally and publish it with few commands.
Let's write your first code for Cloudflare Workers with Hono.
2022-01-02 14:46:36 +01:00
### 1. Install Wrangler
Install Cloudflare Command Line "[Wrangler](https://github.com/cloudflare/wrangler)"
```sh
2022-01-08 21:30:50 +01:00
npm i @cloudflare/wrangler -g
2022-01-02 14:46:36 +01:00
```
### 2. `npm init`
Make npm skeleton directory.
```sh
2022-01-08 21:30:50 +01:00
mkdir hono-example
2022-01-12 18:16:05 +01:00
cd hono-example
2022-01-08 21:30:50 +01:00
npm init -y
2022-01-02 14:46:36 +01:00
```
### 3. `wrangler init`
Init as a wrangler project.
```sh
2022-01-08 21:30:50 +01:00
wrangler init
2022-01-02 14:46:36 +01:00
```
### 4. `npm install hono`
2022-01-05 21:11:37 +01:00
Install `hono` from npm registry.
2022-01-02 14:46:36 +01:00
2022-01-08 21:30:50 +01:00
```sh
npm i hono
2022-01-02 14:46:36 +01:00
```
### 5. Write your app
2022-01-08 01:48:22 +01:00
Only 4 lines!!
2022-01-02 14:46:36 +01:00
```js
2022-01-09 13:10:12 +01:00
import { Hono } from 'hono'
2022-01-02 14:46:36 +01:00
const app = new Hono()
2022-01-05 21:11:37 +01:00
app.get('/', (c) => c.text('Hello! Hono!'))
2022-01-02 14:46:36 +01:00
app.fire()
```
2022-01-05 21:11:37 +01:00
### 6. Run
2022-01-02 14:46:36 +01:00
2022-01-08 01:48:22 +01:00
Run the development server locally. Then, access like `http://127.0.0.1:8787/` in your Web browser.
2022-01-02 14:46:36 +01:00
```sh
2022-01-08 21:30:50 +01:00
wrangler dev
2022-01-02 14:46:36 +01:00
```
2022-02-09 15:14:44 +01:00
### 7. Publish
2022-01-05 21:11:37 +01:00
Deploy to Cloudflare. That's all!
```sh
2022-01-08 21:30:50 +01:00
wrangler publish
2022-01-05 21:11:37 +01:00
```
2021-12-14 21:31:37 +01:00
## Related projects
2022-01-08 22:05:04 +01:00
Implementation of the router is inspired by [goblin ](https://github.com/bmf-san/goblin ). API design is inspired by [express ](https://github.com/expressjs/express ) and [koa ](https://github.com/koajs/koa ). [itty-router ](https://github.com/kwhitley/itty-router ), [Sunder ](https://github.com/SunderJS/sunder ), and [worktop ](https://github.com/lukeed/worktop ) are the other routers or frameworks for Cloudflare Workers.
2022-01-05 21:11:37 +01:00
2021-12-20 23:34:38 +01:00
- express < https: // github . com / expressjs / express >
2022-01-05 21:11:37 +01:00
- koa < https: // github . com / koajs / koa >
2021-12-17 07:53:31 +01:00
- itty-router < https: // github . com / kwhitley / itty-router >
2021-12-20 03:58:40 +01:00
- Sunder < https: // github . com / SunderJS / sunder >
- goblin < https: // github . com / bmf-san / goblin >
2022-01-08 22:05:04 +01:00
- worktop < https: // github . com / lukeed / worktop >
2021-12-14 21:31:37 +01:00
2022-01-05 21:11:37 +01:00
## Contributing
Contributions Welcome! You can contribute by the following way:
- Write or fix documents
- Write code of middleware
- Fix bugs
- Refactor the code
2022-01-08 01:48:22 +01:00
- etc.
2022-01-05 21:11:37 +01:00
2022-01-08 01:48:22 +01:00
If you can, let's make Hono together!
2022-01-05 21:11:37 +01:00
2021-12-14 20:26:22 +01:00
## Author
Yusuke Wada < https: / / github . com / yusukebe >
## License
2022-01-07 09:34:12 +01:00
Distributed under the MIT License. See [LICENSE ](LICENSE ) for more information.