2021-12-17 07:53:31 +01:00
# Hono
2021-12-14 21:17:56 +01:00
2021-12-17 07:53:31 +01:00
Hono [炎] - Tiny web framework for Cloudflare Workers and others.
```js
2022-01-01 07:29:41 +01:00
const { Hono } = require('hono')
const app = new Hono()
2021-12-17 07:53:31 +01:00
2022-01-05 21:11:37 +01:00
app.get('/', (c) => c.text('Hono!!'))
2021-12-17 07:53:31 +01:00
app.fire()
```
2022-01-05 21:11:37 +01:00
Hono[炎] - _**means flame🔥 in Japanese**_ - is small, fast and simple web flamework for a Service Workers API based serverless service such as **Cloudflare Workers** and **Fastly Compute@Edge** . Because Hono does not depend on any NPM packages, it's easy to install, setup, and deploy. Although Hono has a router, context object, and middleware including builtin. Mostly web application like a Web API can be made.
In the case of Cloudflare Workers, there are `wrangler` and `miniflare` .You can develop the application locally and publish it with few commands.
It's amazing that the experience of writing code with Hono. Enjoy!
2022-01-02 14:46:36 +01:00
2021-12-17 07:53:31 +01:00
## Feature
2022-01-05 21:11:37 +01:00
- **Fast** - the router is implemented with Trie-Tree structure.
- **Tiny** - zero dependencies, using Web standard API.
- **Flexible** - you can make your own middleware.
- **Easy** - simple API, builtin middleware, and written in TypeScript.
- **Optimized** - for Cloudflare Workers or Fastly Compute@Edge.
2021-12-14 21:17:56 +01:00
2021-12-27 17:34:09 +01:00
## Benchmark
2022-01-05 21:11:37 +01:00
**Hono is fastest** compared to other routers for Cloudflare Workers.
2022-01-05 10:41:29 +01:00
2021-12-27 17:34:09 +01:00
```
2022-01-05 10:41:29 +01:00
hono x 758,264 ops/sec ±5.41% (75 runs sampled)
itty-router x 158,359 ops/sec ±3.21% (89 runs sampled)
sunder x 297,581 ops/sec ±4.74% (83 runs sampled)
2021-12-27 17:34:09 +01:00
Fastest is hono
2022-01-05 10:41:29 +01:00
✨ Done in 42.84s.
2021-12-27 17:34:09 +01:00
```
2022-01-05 21:11:37 +01:00
## Hono in 1 minute
Below is demonstration to create an application of Cloudflare Workers with Hono.
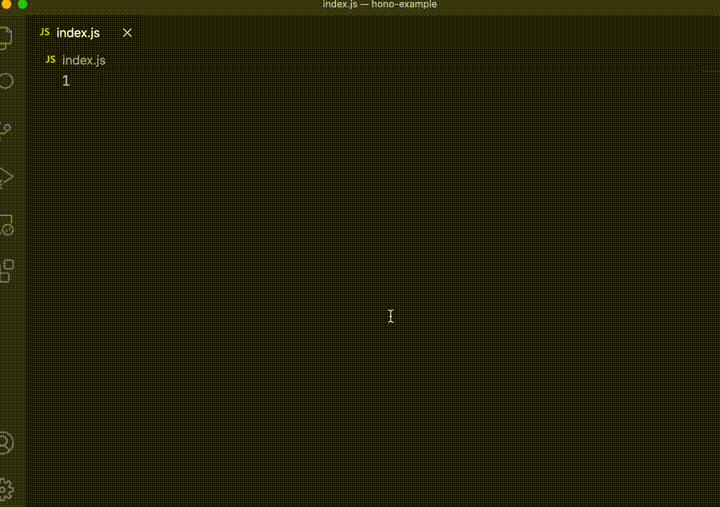
2021-12-14 21:17:56 +01:00
## Install
2022-01-05 21:11:37 +01:00
You can install from npm public registry:
2021-12-20 23:34:38 +01:00
```
2021-12-14 21:17:56 +01:00
$ yarn add hono
```
or
```sh
$ npm install hono
```
2021-12-20 03:58:40 +01:00
## Methods
2022-01-05 21:11:37 +01:00
Instance of `Hono` has these methods:
2022-01-05 10:41:29 +01:00
- app.**HTTP_METHOD**(path, handler)
- app.**all**(path, handler)
2021-12-20 03:58:40 +01:00
- app.**route**(path)
2021-12-20 23:34:38 +01:00
- app.**use**(path, middleware)
2022-01-05 21:19:16 +01:00
- app.**fire**()
2021-12-20 03:58:40 +01:00
2021-12-17 07:53:31 +01:00
## Routing
### Basic
2021-12-14 21:17:56 +01:00
2021-12-20 03:58:40 +01:00
`app.HTTP_METHOD`
2021-12-14 21:17:56 +01:00
```js
2021-12-20 23:34:38 +01:00
// HTTP Methods
app.get('/', () => new Response('GET /'))
app.post('/', () => new Response('POST /'))
// Wildcard
2021-12-21 09:37:02 +01:00
app.get('/wild/*/card', () => {
2021-12-21 10:37:24 +01:00
return new Response('GET /wild/*/card')
2021-12-20 23:34:38 +01:00
})
2021-12-17 07:53:31 +01:00
```
2021-12-14 21:17:56 +01:00
2021-12-20 03:58:40 +01:00
`app.all`
```js
2021-12-20 23:34:38 +01:00
// Any HTTP methods
2021-12-22 20:24:14 +01:00
app.all('/hello', () => new Response('ALL Method /hello'))
2021-12-20 03:58:40 +01:00
```
2021-12-17 07:53:31 +01:00
### Named Parameter
2021-12-14 21:17:56 +01:00
2021-12-17 07:53:31 +01:00
```js
2021-12-21 09:37:02 +01:00
app.get('/user/:name', (c) => {
const name = c.req.params('name')
2021-12-17 07:53:31 +01:00
...
})
```
### Regexp
```js
2021-12-21 09:37:02 +01:00
app.get('/post/:date{[0-9]+}/:title{[a-z]+}', (c) => {
const date = c.req.params('date')
const title = c.req.params('title')
2021-12-17 07:53:31 +01:00
...
2021-12-14 21:17:56 +01:00
```
2021-12-17 07:53:31 +01:00
### Chained Route
```js
app
.route('/api/book')
2021-12-21 09:37:02 +01:00
.get(() => {...})
.post(() => {...})
.put(() => {...})
2021-12-17 07:53:31 +01:00
```
2021-12-14 20:26:22 +01:00
2022-01-05 21:11:37 +01:00
## async/await
2022-01-03 10:11:46 +01:00
```js
app.get('/fetch-url', async () => {
const response = await fetch('https://example.com/')
return new Response(`Status is ${response.status}`)
})
```
2021-12-20 03:58:40 +01:00
## Middleware
2022-01-01 08:22:35 +01:00
### Builtin Middleware
```js
const { Hono, Middleware } = require('hono')
...
2022-01-05 10:41:29 +01:00
app.use('*', Middleware.poweredBy())
app.use('*', Middleware.logger())
2022-01-01 08:22:35 +01:00
```
2022-01-05 21:11:37 +01:00
Available builtin middleware are listed on [src/middleware ](https://github.com/yusukebe/hono/tree/master/src/middleware ) directory.
2022-01-01 08:22:35 +01:00
### Custom Middleware
2022-01-05 21:11:37 +01:00
You can write your own middleware:
2021-12-20 03:58:40 +01:00
```js
2022-01-05 10:41:29 +01:00
// Custom logger
app.use('*', async (c, next) => {
2021-12-21 09:37:02 +01:00
console.log(`[${c.req.method}] ${c.req.url}`)
2022-01-03 10:11:46 +01:00
await next()
2022-01-05 10:41:29 +01:00
})
2021-12-20 03:58:40 +01:00
2022-01-05 10:41:29 +01:00
// Add custom header
app.use('/message/*', async (c, next) => {
2022-01-03 10:11:46 +01:00
await next()
await c.res.headers.add('x-message', 'This is middleware!')
2022-01-05 10:41:29 +01:00
})
2021-12-20 18:35:03 +01:00
app.get('/message/hello', () => 'Hello Middleware!')
2021-12-20 03:58:40 +01:00
```
2022-01-01 14:54:29 +01:00
### Custom 404 Response
2022-01-05 21:11:37 +01:00
If you want to customize 404 Not Found response:
2022-01-01 14:54:29 +01:00
```js
2022-01-05 10:41:29 +01:00
app.use('*', async (c, next) => {
2022-01-03 10:11:46 +01:00
await next()
2022-01-01 14:54:29 +01:00
if (c.res.status === 404) {
c.res = new Response('Custom 404 Not Found', { status: 404 })
}
2022-01-05 10:41:29 +01:00
})
2022-01-01 14:54:29 +01:00
```
2022-01-03 10:11:46 +01:00
### Complex Pattern
2022-01-05 21:11:37 +01:00
You can also do this:
2022-01-03 10:11:46 +01:00
```js
2022-01-05 10:41:29 +01:00
// Output response time
2022-01-03 10:11:46 +01:00
app.use('*', async (c, next) => {
await next()
const responseTime = await c.res.headers.get('X-Response-Time')
console.log(`X-Response-Time: ${responseTime}`)
})
// Add X-Response-Time header
app.use('*', async (c, next) => {
const start = Date.now()
await next()
const ms = Date.now() - start
await c.res.headers.append('X-Response-Time', `${ms}ms` )
})
```
2021-12-21 09:37:02 +01:00
## Context
2022-01-05 21:11:37 +01:00
To handle Request and Reponse easily, you can use Context object:
### c.req
2021-12-21 09:37:02 +01:00
```js
2022-01-05 10:41:29 +01:00
// Get Request object
2021-12-21 09:37:02 +01:00
app.get('/hello', (c) => {
2022-01-01 16:32:01 +01:00
const userAgent = c.req.headers.get('User-Agent')
2021-12-21 09:37:02 +01:00
...
})
2022-01-05 10:41:29 +01:00
// Query params
app.get('/search', (c) => {
const query = c.req.query('q')
...
})
// Captured params
app.get('/entry/:id', (c) => {
const id = c.req.params('id')
...
})
2021-12-21 09:37:02 +01:00
```
2022-01-05 21:11:37 +01:00
### c.res
2021-12-21 09:37:02 +01:00
```js
2022-01-05 10:41:29 +01:00
// Response object
2022-01-01 16:29:03 +01:00
app.use('/', (c, next) => {
2021-12-21 09:37:02 +01:00
next()
2022-01-01 16:29:03 +01:00
c.res.headers.append('X-Debug', 'Debug message')
2021-12-21 09:37:02 +01:00
})
```
2022-01-05 21:11:37 +01:00
### c.text()
Render text as `Content-Type:text/plain` :
2021-12-20 03:58:40 +01:00
```js
2022-01-05 10:41:29 +01:00
app.get('/say', (c) => {
return c.text('Hello!')
2021-12-20 03:58:40 +01:00
})
```
2022-01-05 21:11:37 +01:00
### c.json()
Render text as `Content-Type:application/json` :
2022-01-05 19:22:53 +01:00
```js
app.get('/api', (c) => {
return c.json({ message: 'Hello!' })
})
```
2022-01-05 21:19:16 +01:00
## fire
`app.fire()` do:
```js
addEventListener('fetch', (event) => {
event.respondWith(this.handleEvent(event))
})
```
2022-01-05 21:11:37 +01:00
## Cloudflare Workers with Hono
2022-01-02 14:46:36 +01:00
2022-01-05 21:11:37 +01:00
Write your first code for Cloudflare Workers with Hono.
2022-01-02 14:46:36 +01:00
### 1. Install Wrangler
Install Cloudflare Command Line "[Wrangler](https://github.com/cloudflare/wrangler)"
```sh
$ npm i @cloudflare/wrangler -g
```
### 2. `npm init`
Make npm skeleton directory.
```sh
$ mkdir hono-example
$ ch hono-example
$ npm init -y
```
### 3. `wrangler init`
Init as a wrangler project.
```sh
$ wrangler init
```
### 4. `npm install hono`
2022-01-05 21:11:37 +01:00
Install `hono` from npm registry.
2022-01-02 14:46:36 +01:00
```
$ npm i hono
```
### 5. Write your app
Only 4 line!!
```js
const { Hono } = require('hono')
const app = new Hono()
2022-01-05 21:11:37 +01:00
app.get('/', (c) => c.text('Hello! Hono!'))
2022-01-02 14:46:36 +01:00
app.fire()
```
2022-01-05 21:11:37 +01:00
### 6. Run
2022-01-02 14:46:36 +01:00
Run the development server locally.
```sh
$ wrangler dev
```
2022-01-05 21:11:37 +01:00
### Publish
Deploy to Cloudflare. That's all!
```sh
$ wrangler publish
```
2021-12-14 21:31:37 +01:00
## Related projects
2022-01-05 21:11:37 +01:00
Implementation of the router is inspired by [goblin ](https://github.com/bmf-san/goblin ). API design is inspired by [express ](https://github.com/expressjs/express ) and [koa ](https://github.com/koajs/koa ). [itty-router ](https://github.com/kwhitley/itty-router ) and [Sunder ](https://github.com/SunderJS/sunder ) are the other routers or frameworks for Cloudflare Workers.
2021-12-20 23:34:38 +01:00
- express < https: // github . com / expressjs / express >
2022-01-05 21:11:37 +01:00
- koa < https: // github . com / koajs / koa >
2021-12-17 07:53:31 +01:00
- itty-router < https: // github . com / kwhitley / itty-router >
2021-12-20 03:58:40 +01:00
- Sunder < https: // github . com / SunderJS / sunder >
- goblin < https: // github . com / bmf-san / goblin >
2021-12-14 21:31:37 +01:00
2022-01-05 21:11:37 +01:00
## Contributing
Contributions Welcome! You can contribute by the following way:
- Write or fix documents
- Write code of middleware
- Fix bugs
- Refactor the code
If you can, please!
2021-12-14 20:26:22 +01:00
## Author
Yusuke Wada < https: / / github . com / yusukebe >
## License
2022-01-05 21:11:37 +01:00
Distributed under the MIT License. See [LICENSE ](LISENCE ) for more information.