mirror of
https://github.com/garraflavatra/trmclr.git
synced 2025-04-20 04:11:02 +00:00
Compare commits
No commits in common. "main" and "v1.0.0" have entirely different histories.
28
.github/workflows/ci.yml
vendored
28
.github/workflows/ci.yml
vendored
@ -1,28 +0,0 @@
|
||||
name: CI
|
||||
|
||||
on:
|
||||
push:
|
||||
branches: [ "main" ]
|
||||
pull_request:
|
||||
branches: [ "main" ]
|
||||
|
||||
jobs:
|
||||
build:
|
||||
runs-on: ubuntu-latest
|
||||
|
||||
strategy:
|
||||
fail-fast: false
|
||||
matrix:
|
||||
node-version: [16.x, 18.x]
|
||||
|
||||
steps:
|
||||
- uses: actions/checkout@v4
|
||||
- name: Use Node.js ${{ matrix.node-version }}
|
||||
uses: actions/setup-node@v3
|
||||
with:
|
||||
node-version: ${{ matrix.node-version }}
|
||||
cache: 'npm'
|
||||
|
||||
- run: npm ci
|
||||
- run: npm run lint
|
||||
- run: npm run build
|
51
README.md
51
README.md
@ -1,51 +0,0 @@
|
||||
# trmclr
|
||||
|
||||
[](https://www.npmjs.com/package/trmclr)
|
||||
|
||||
Easy terminal colors for browsers, [Node.js](https://nodejs.org), [Deno](https://deno.com), [Bun](https://bun.sh), and probably some other environments.
|
||||
|
||||
- Comes with 45 colors and helpers.
|
||||
- Respects [`NO_COLOR`](https://no-color.org/).
|
||||
- No dependencies.
|
||||
- Tree-shakeable.
|
||||
|
||||
## Colors and helpers
|
||||
|
||||
bgBlack, bgBlue, bgBrightBlack, bgBrightBlue, bgBrightCyan, bgBrightGreen, bgBrightMagenta, bgBrightRed, bgBrightWhite, bgBrightYellow, bgCyan, bgGreen, bgMagenta, bgRed, bgRgb24, bgRgb8, bgWhite, bgYellow, black, blue, bold, brightBlack, brightBlue, brightCyan, brightGreen, brightMagenta, brightRed, brightWhite, brightYellow, cyan, dim, getColorEnabled, gray, green, hidden, inverse, italic, magenta, red, reset, strikethrough, stripColor, underline, white, yellow
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
import { cyan, bgMagenta } from 'trmclr';
|
||||
|
||||
console.log(cyan(bgMagenta('Some text')));
|
||||
```
|
||||
|
||||
### Enable or disable color
|
||||
|
||||
```js
|
||||
import { getColorEnabled, setColorEnabled } from 'trmclr';
|
||||
|
||||
setColorEnabled(true);
|
||||
setColorEnabled(false);
|
||||
|
||||
getColorEnabled(); // → false
|
||||
```
|
||||
|
||||
### Remove colors and decorations
|
||||
|
||||
```js
|
||||
import { cyan, stripColor } from 'trmclr';
|
||||
|
||||
stripColor(cyan('Hi')); // → uncolored "Hi"
|
||||
```
|
||||
|
||||
## License
|
||||
|
||||
(c) 2022-2024 [Romein van Buren](mailto:romein@vburen.nl). Licensed under the MIT license.
|
||||
|
||||
For the full copyright and license information, please see the [`LICENSE.md`](./LICENSE.md) file that was distributed with this source code.
|
||||
|
||||
Original implementation: (c) 2018-2022 [the Deno authors](https://github.com/denoland/std/blob/0.159.0/fmt/colors.ts).
|
||||
|
||||
[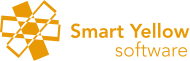](https://www.smartyellow.nl)
|
543
index.mjs
543
index.mjs
@ -1,543 +0,0 @@
|
||||
/* global globalThis */
|
||||
|
||||
/**
|
||||
* @typedef Color
|
||||
* @property {string} open
|
||||
* @property {string} close
|
||||
* @property {RegExp} regexp
|
||||
*/
|
||||
|
||||
/**
|
||||
* @typedef RGB
|
||||
* @property {number} r
|
||||
* @property {number} g
|
||||
* @property {number} b
|
||||
*/
|
||||
|
||||
/**
|
||||
* True if `NO_COLOR` is set.
|
||||
* @see https://no-color.org/
|
||||
*/
|
||||
// @ts-expect-error Depends on environment.
|
||||
export const noColor = !!globalThis.process?.env?.NO_COLOR || !!globalThis.Deno?.noColor;
|
||||
|
||||
let enabled = !noColor;
|
||||
|
||||
/**
|
||||
* Enable or disable terminal coloring.
|
||||
* @param {boolean} value Whether to enable color output.
|
||||
*/
|
||||
export function setColorEnabled(value) {
|
||||
enabled = !!value;
|
||||
}
|
||||
|
||||
export function getColorEnabled() {
|
||||
return enabled;
|
||||
}
|
||||
|
||||
/**
|
||||
* @private
|
||||
* @param {number[]} open
|
||||
* @param {number} close
|
||||
* @returns {Code}
|
||||
*/
|
||||
function code(open, close) {
|
||||
return {
|
||||
open: `\x1b[${open.join(';')}m`,
|
||||
close: `\x1b[${close}m`,
|
||||
regexp: new RegExp(`\\x1b\\[${close}m`, 'g'),
|
||||
};
|
||||
}
|
||||
|
||||
/**
|
||||
* @private
|
||||
* @param {string} str
|
||||
* @param {Code} code
|
||||
* @returns {string}
|
||||
*/
|
||||
function run(str, code) {
|
||||
return enabled ? `${code.open}${str.replace(code.regexp, code.open)}${code.close}` : str;
|
||||
}
|
||||
|
||||
/**
|
||||
* Reset color.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function reset(str) {
|
||||
return run(str, code([ 0 ], 0));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str bold.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bold(str) {
|
||||
return run(str, code([ 1 ], 22));
|
||||
}
|
||||
|
||||
/**
|
||||
* Dim str.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function dim(str) {
|
||||
return run(str, code([ 2 ], 22));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str italic.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function italic(str) {
|
||||
return run(str, code([ 3 ], 23));
|
||||
}
|
||||
|
||||
/**
|
||||
* Underline str.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function underline(str) {
|
||||
return run(str, code([ 4 ], 24));
|
||||
}
|
||||
|
||||
/**
|
||||
* Invert the color of str.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function inverse(str) {
|
||||
return run(str, code([ 7 ], 27));
|
||||
}
|
||||
|
||||
/**
|
||||
* Hide str.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function hidden(str) {
|
||||
return run(str, code([ 8 ], 28));
|
||||
}
|
||||
|
||||
/**
|
||||
* Strike str.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function strikethrough(str) {
|
||||
return run(str, code([ 9 ], 29));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str black.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function black(str) {
|
||||
return run(str, code([ 30 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str red.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function red(str) {
|
||||
return run(str, code([ 31 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str green.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function green(str) {
|
||||
return run(str, code([ 32 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str yellow.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function yellow(str) {
|
||||
return run(str, code([ 33 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str blue.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function blue(str) {
|
||||
return run(str, code([ 34 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str magenta.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function magenta(str) {
|
||||
return run(str, code([ 35 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str cyan.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function cyan(str) {
|
||||
return run(str, code([ 36 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str white.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function white(str) {
|
||||
return run(str, code([ 37 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str gray.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function gray(str) {
|
||||
return brightBlack(str);
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str bright black.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function brightBlack(str) {
|
||||
return run(str, code([ 90 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str bright red.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function brightRed(str) {
|
||||
return run(str, code([ 91 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str bright rgeen.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function brightGreen(str) {
|
||||
return run(str, code([ 92 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str bright yellow.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function brightYellow(str) {
|
||||
return run(str, code([ 93 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str bright blue.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function brightBlue(str) {
|
||||
return run(str, code([ 94 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str bright magenta.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function brightMagenta(str) {
|
||||
return run(str, code([ 95 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str bright cyan.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function brightCyan(str) {
|
||||
return run(str, code([ 96 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Make str bright white.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function brightWhite(str) {
|
||||
return run(str, code([ 97 ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a black background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgBlack(str) {
|
||||
return run(str, code([ 40 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a red background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgRed(str) {
|
||||
return run(str, code([ 41 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a green background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgGreen(str) {
|
||||
return run(str, code([ 42 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a yellow background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgYellow(str) {
|
||||
return run(str, code([ 43 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a blue background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgBlue(str) {
|
||||
return run(str, code([ 44 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a magenta background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgMagenta(str) {
|
||||
return run(str, code([ 45 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a cyan background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgCyan(str) {
|
||||
return run(str, code([ 46 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a white background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgWhite(str) {
|
||||
return run(str, code([ 47 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a bright black background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgBrightBlack(str) {
|
||||
return run(str, code([ 100 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a bright red background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgBrightRed(str) {
|
||||
return run(str, code([ 101 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a bright green background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgBrightGreen(str) {
|
||||
return run(str, code([ 102 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a bright yellow background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgBrightYellow(str) {
|
||||
return run(str, code([ 103 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a bright blue background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgBrightBlue(str) {
|
||||
return run(str, code([ 104 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a bright magenta background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgBrightMagenta(str) {
|
||||
return run(str, code([ 105 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a bright cyan background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgBrightCyan(str) {
|
||||
return run(str, code([ 106 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Give str a bright white background.
|
||||
* @param {string} str String to color.
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgBrightWhite(str) {
|
||||
return run(str, code([ 107 ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* @private
|
||||
* @param {number} n
|
||||
* @param {number} max
|
||||
* @param {number} min
|
||||
* @returns {number}
|
||||
*/
|
||||
function clampAndTruncate(n, max = 255, min = 0) {
|
||||
return Math.trunc(Math.max(Math.min(n, max), min));
|
||||
}
|
||||
|
||||
/**
|
||||
* Set str's color using paletted 8-bit colors.
|
||||
* @see https://en.wikipedia.org/wiki/ANSI_escape_code#8-bit
|
||||
*
|
||||
* @param {string} str String to color.
|
||||
* @param {number} color 8-bit RGB color
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function rgb8(str, color) {
|
||||
return run(str, code([ 38, 5, clampAndTruncate(color) ], 39));
|
||||
}
|
||||
|
||||
/**
|
||||
* Set str's background color using paletted 8-bit colors.
|
||||
* @see https://en.wikipedia.org/wiki/ANSI_escape_code#8-bit
|
||||
*
|
||||
* @param {string} str String to color.
|
||||
* @param {number} color 8-bit RGB color
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgRgb8(str, color) {
|
||||
return run(str, code([ 48, 5, clampAndTruncate(color) ], 49));
|
||||
}
|
||||
|
||||
/**
|
||||
* Set str's color using paletted 8-bit colors.
|
||||
* @see https://en.wikipedia.org/wiki/ANSI_escape_code#8-bit
|
||||
*
|
||||
* @param {string} str String to color.
|
||||
* @param {number | RGB} color 8-bit RGB color
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function rgb24(str, color) {
|
||||
if (typeof color === 'number') {
|
||||
return run(
|
||||
str,
|
||||
code([ 38, 2, (color >> 16) & 0xff, (color >> 8) & 0xff, color & 0xff ], 39)
|
||||
);
|
||||
}
|
||||
return run(
|
||||
str,
|
||||
code(
|
||||
[
|
||||
38,
|
||||
2,
|
||||
clampAndTruncate(color.r),
|
||||
clampAndTruncate(color.g),
|
||||
clampAndTruncate(color.b),
|
||||
],
|
||||
39
|
||||
)
|
||||
);
|
||||
}
|
||||
|
||||
/**
|
||||
* Set str's background using paletted 24-bit colors.
|
||||
* @see https://en.wikipedia.org/wiki/ANSI_escape_code#24-bit
|
||||
*
|
||||
* @param {string} str String to color.
|
||||
* @param {number | RGB} color 24-bit RGB color
|
||||
* @returns Colored string.
|
||||
*/
|
||||
export function bgRgb24(str, color) {
|
||||
if (typeof color === 'number') {
|
||||
return run(
|
||||
str,
|
||||
code([ 48, 2, (color >> 16) & 0xff, (color >> 8) & 0xff, color & 0xff ], 49)
|
||||
);
|
||||
}
|
||||
return run(
|
||||
str,
|
||||
code(
|
||||
[
|
||||
48,
|
||||
2,
|
||||
clampAndTruncate(color.r),
|
||||
clampAndTruncate(color.g),
|
||||
clampAndTruncate(color.b),
|
||||
],
|
||||
49
|
||||
)
|
||||
);
|
||||
}
|
||||
|
||||
// https://github.com/chalk/ansi-regex/blob/02fa893d619d3da85411acc8fd4e2eea0e95a9d9/index.js
|
||||
const ANSI_PATTERN = new RegExp(
|
||||
[
|
||||
'[\\u001B\\u009B][[\\]()#;?]*(?:(?:(?:(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]+)*|[a-zA-Z\\d]+(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]*)*)?\\u0007)',
|
||||
'(?:(?:\\d{1,4}(?:;\\d{0,4})*)?[\\dA-PR-TZcf-nq-uy=><~]))',
|
||||
].join('|'),
|
||||
'g'
|
||||
);
|
||||
|
||||
/**
|
||||
* Remove all colors and decorations from str.
|
||||
*
|
||||
* @param {string} str String to uncolor.
|
||||
* @returns Uncolored string.
|
||||
*/
|
||||
export function stripColor(str) {
|
||||
return str.replace(ANSI_PATTERN, '');
|
||||
}
|
@ -1,4 +1,4 @@
|
||||
Copyright © 2022-2024 Romein van Buren
|
||||
Copyright © 2022 Romein van Buren
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the “Software”), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
|
||||
|
5651
package-lock.json
generated
5651
package-lock.json
generated
File diff suppressed because it is too large
Load Diff
41
package.json
41
package.json
@ -1,17 +1,16 @@
|
||||
{
|
||||
"name": "trmclr",
|
||||
"version": "1.1.0",
|
||||
"version": "1.0.0",
|
||||
"description": "Terminal colors but easier",
|
||||
"author": "Romein van Buren <romein@vburen.nl>",
|
||||
"license": "MIT",
|
||||
"main": "dist/index.cjs",
|
||||
"module": "index.mjs",
|
||||
"types": "dist/index.d.mts",
|
||||
"type": "module",
|
||||
"main": "dist/index.js",
|
||||
"types": "dist/index.d.ts",
|
||||
"files": [
|
||||
"index.mjs",
|
||||
"dist"
|
||||
"dist",
|
||||
"src"
|
||||
],
|
||||
"scripts": {
|
||||
"build": "tsup src/index.ts --format cjs --dts"
|
||||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "git+https://github.com/garraflavatra/trmclr.git"
|
||||
@ -22,29 +21,15 @@
|
||||
"terminal",
|
||||
"console"
|
||||
],
|
||||
"author": "Romein van Buren <romein@vburen.nl>",
|
||||
"license": "MIT",
|
||||
"bugs": {
|
||||
"url": "https://github.com/garraflavatra/trmclr/issues"
|
||||
},
|
||||
"homepage": "https://github.com/garraflavatra/trmclr#readme",
|
||||
"scripts": {
|
||||
"build": "rm -rf dist && tsc && rollup index.mjs -f umd -n trmclr -o dist/index.cjs",
|
||||
"lint": "eslint index.mjs"
|
||||
},
|
||||
"devDependencies": {
|
||||
"@garraflavatra/yeslint": "^1.2.0",
|
||||
"@types/node": "^22.1.0",
|
||||
"@typescript-eslint/eslint-plugin": "^6.21.0",
|
||||
"eslint": "^8.57.0",
|
||||
"rollup": "^4.20.0",
|
||||
"typescript": "^5.5.4"
|
||||
},
|
||||
"eslintConfig": {
|
||||
"extends": "./node_modules/@garraflavatra/yeslint/configs/generic.js",
|
||||
"ignorePatterns": [
|
||||
"dist"
|
||||
],
|
||||
"parserOptions": {
|
||||
"sourceType": "module"
|
||||
}
|
||||
"@types/node": "^18.8.3",
|
||||
"tsup": "^6.2.3",
|
||||
"typescript": "^4.8.4"
|
||||
}
|
||||
}
|
||||
|
28
readme.md
Normal file
28
readme.md
Normal file
@ -0,0 +1,28 @@
|
||||
# trmclr
|
||||
|
||||
- Easy terminal colors, ported from the Deno standard library.
|
||||
- Supports Node.js, browsers, Deno, and probably some other environments.
|
||||
- Comes with 45 colors and helpers.
|
||||
- Respects [`NO_COLOR`](http://no-color.org/).
|
||||
- No dependencies.
|
||||
- Allows for tree-shaking.
|
||||
|
||||
## Colors and helpers
|
||||
|
||||
bgBlack, bgBlue, bgBrightBlack, bgBrightBlue, bgBrightCyan, bgBrightGreen, bgBrightMagenta, bgBrightRed, bgBrightWhite, bgBrightYellow, bgCyan, bgGreen, bgMagenta, bgRed, bgRgb24, bgRgb8, bgWhite, bgYellow, black, blue, bold, brightBlack, brightBlue, brightCyan, brightGreen, brightMagenta, brightRed, brightWhite, brightYellow, cyan, dim, getColorEnabled, gray, green, hidden, inverse, italic, magenta, red, reset, strikethrough, stripColor, underline, white, yellow
|
||||
|
||||
## Usage
|
||||
|
||||
```js
|
||||
import { cyan, bgMagenta } from 'trmcrl';
|
||||
|
||||
console.log(cyan(bgMagenta('Some text')));
|
||||
```
|
||||
|
||||
## Enable or disable color
|
||||
|
||||
By using `setColorEnabled(true)` or `setColorEnabled(false)` you can respectively enable and disable color output. Use `getColorEnabled()` to retrieve the current state.
|
||||
|
||||
## License
|
||||
|
||||
MIT
|
290
src/index.ts
Normal file
290
src/index.ts
Normal file
@ -0,0 +1,290 @@
|
||||
// This has been vendored from the Deno standard library and ported to Node.js.
|
||||
// https://github.com/denoland/deno_std/blob/0.159.0/fmt/colors.ts
|
||||
|
||||
declare const process:
|
||||
| {
|
||||
env?: {
|
||||
NO_COLOR?: boolean;
|
||||
};
|
||||
}
|
||||
| undefined;
|
||||
|
||||
declare const Deno:
|
||||
| {
|
||||
noColor: boolean;
|
||||
}
|
||||
| undefined;
|
||||
|
||||
interface Code {
|
||||
open: string;
|
||||
close: string;
|
||||
regexp: RegExp;
|
||||
}
|
||||
|
||||
interface Rgb {
|
||||
r: number;
|
||||
g: number;
|
||||
b: number;
|
||||
}
|
||||
|
||||
const noColor = !!process?.env?.NO_COLOR || !!Deno?.noColor;
|
||||
let enabled = !noColor;
|
||||
|
||||
export function setColorEnabled(value: boolean) {
|
||||
if (noColor) {
|
||||
return;
|
||||
}
|
||||
|
||||
enabled = value;
|
||||
}
|
||||
|
||||
export function getColorEnabled(): boolean {
|
||||
return enabled;
|
||||
}
|
||||
|
||||
function code(open: number[], close: number): Code {
|
||||
return {
|
||||
open: `\x1b[${open.join(';')}m`,
|
||||
close: `\x1b[${close}m`,
|
||||
regexp: new RegExp(`\\x1b\\[${close}m`, 'g'),
|
||||
};
|
||||
}
|
||||
|
||||
function run(str: string, code: Code): string {
|
||||
return enabled
|
||||
? `${code.open}${str.replace(code.regexp, code.open)}${code.close}`
|
||||
: str;
|
||||
}
|
||||
|
||||
export function reset(str: string): string {
|
||||
return run(str, code([0], 0));
|
||||
}
|
||||
|
||||
export function bold(str: string): string {
|
||||
return run(str, code([1], 22));
|
||||
}
|
||||
|
||||
export function dim(str: string): string {
|
||||
return run(str, code([2], 22));
|
||||
}
|
||||
|
||||
export function italic(str: string): string {
|
||||
return run(str, code([3], 23));
|
||||
}
|
||||
|
||||
export function underline(str: string): string {
|
||||
return run(str, code([4], 24));
|
||||
}
|
||||
|
||||
export function inverse(str: string): string {
|
||||
return run(str, code([7], 27));
|
||||
}
|
||||
|
||||
export function hidden(str: string): string {
|
||||
return run(str, code([8], 28));
|
||||
}
|
||||
|
||||
export function strikethrough(str: string): string {
|
||||
return run(str, code([9], 29));
|
||||
}
|
||||
|
||||
export function black(str: string): string {
|
||||
return run(str, code([30], 39));
|
||||
}
|
||||
|
||||
export function red(str: string): string {
|
||||
return run(str, code([31], 39));
|
||||
}
|
||||
|
||||
export function green(str: string): string {
|
||||
return run(str, code([32], 39));
|
||||
}
|
||||
|
||||
export function yellow(str: string): string {
|
||||
return run(str, code([33], 39));
|
||||
}
|
||||
|
||||
export function blue(str: string): string {
|
||||
return run(str, code([34], 39));
|
||||
}
|
||||
|
||||
export function magenta(str: string): string {
|
||||
return run(str, code([35], 39));
|
||||
}
|
||||
|
||||
export function cyan(str: string): string {
|
||||
return run(str, code([36], 39));
|
||||
}
|
||||
|
||||
export function white(str: string): string {
|
||||
return run(str, code([37], 39));
|
||||
}
|
||||
|
||||
export function gray(str: string): string {
|
||||
return brightBlack(str);
|
||||
}
|
||||
|
||||
export function brightBlack(str: string): string {
|
||||
return run(str, code([90], 39));
|
||||
}
|
||||
|
||||
export function brightRed(str: string): string {
|
||||
return run(str, code([91], 39));
|
||||
}
|
||||
|
||||
export function brightGreen(str: string): string {
|
||||
return run(str, code([92], 39));
|
||||
}
|
||||
|
||||
export function brightYellow(str: string): string {
|
||||
return run(str, code([93], 39));
|
||||
}
|
||||
|
||||
export function brightBlue(str: string): string {
|
||||
return run(str, code([94], 39));
|
||||
}
|
||||
|
||||
export function brightMagenta(str: string): string {
|
||||
return run(str, code([95], 39));
|
||||
}
|
||||
|
||||
export function brightCyan(str: string): string {
|
||||
return run(str, code([96], 39));
|
||||
}
|
||||
|
||||
export function brightWhite(str: string): string {
|
||||
return run(str, code([97], 39));
|
||||
}
|
||||
|
||||
export function bgBlack(str: string): string {
|
||||
return run(str, code([40], 49));
|
||||
}
|
||||
|
||||
export function bgRed(str: string): string {
|
||||
return run(str, code([41], 49));
|
||||
}
|
||||
|
||||
export function bgGreen(str: string): string {
|
||||
return run(str, code([42], 49));
|
||||
}
|
||||
|
||||
export function bgYellow(str: string): string {
|
||||
return run(str, code([43], 49));
|
||||
}
|
||||
|
||||
export function bgBlue(str: string): string {
|
||||
return run(str, code([44], 49));
|
||||
}
|
||||
|
||||
export function bgMagenta(str: string): string {
|
||||
return run(str, code([45], 49));
|
||||
}
|
||||
|
||||
export function bgCyan(str: string): string {
|
||||
return run(str, code([46], 49));
|
||||
}
|
||||
|
||||
export function bgWhite(str: string): string {
|
||||
return run(str, code([47], 49));
|
||||
}
|
||||
|
||||
export function bgBrightBlack(str: string): string {
|
||||
return run(str, code([100], 49));
|
||||
}
|
||||
|
||||
export function bgBrightRed(str: string): string {
|
||||
return run(str, code([101], 49));
|
||||
}
|
||||
|
||||
export function bgBrightGreen(str: string): string {
|
||||
return run(str, code([102], 49));
|
||||
}
|
||||
|
||||
export function bgBrightYellow(str: string): string {
|
||||
return run(str, code([103], 49));
|
||||
}
|
||||
|
||||
export function bgBrightBlue(str: string): string {
|
||||
return run(str, code([104], 49));
|
||||
}
|
||||
|
||||
export function bgBrightMagenta(str: string): string {
|
||||
return run(str, code([105], 49));
|
||||
}
|
||||
|
||||
export function bgBrightCyan(str: string): string {
|
||||
return run(str, code([106], 49));
|
||||
}
|
||||
|
||||
export function bgBrightWhite(str: string): string {
|
||||
return run(str, code([107], 49));
|
||||
}
|
||||
|
||||
function clampAndTruncate(n: number, max = 255, min = 0): number {
|
||||
return Math.trunc(Math.max(Math.min(n, max), min));
|
||||
}
|
||||
|
||||
export function rgb8(str: string, color: number): string {
|
||||
return run(str, code([38, 5, clampAndTruncate(color)], 39));
|
||||
}
|
||||
|
||||
export function bgRgb8(str: string, color: number): string {
|
||||
return run(str, code([48, 5, clampAndTruncate(color)], 49));
|
||||
}
|
||||
|
||||
export function rgb24(str: string, color: number | Rgb): string {
|
||||
if (typeof color === 'number') {
|
||||
return run(
|
||||
str,
|
||||
code([38, 2, (color >> 16) & 0xff, (color >> 8) & 0xff, color & 0xff], 39)
|
||||
);
|
||||
}
|
||||
return run(
|
||||
str,
|
||||
code(
|
||||
[
|
||||
38,
|
||||
2,
|
||||
clampAndTruncate(color.r),
|
||||
clampAndTruncate(color.g),
|
||||
clampAndTruncate(color.b),
|
||||
],
|
||||
39
|
||||
)
|
||||
);
|
||||
}
|
||||
|
||||
export function bgRgb24(str: string, color: number | Rgb): string {
|
||||
if (typeof color === 'number') {
|
||||
return run(
|
||||
str,
|
||||
code([48, 2, (color >> 16) & 0xff, (color >> 8) & 0xff, color & 0xff], 49)
|
||||
);
|
||||
}
|
||||
return run(
|
||||
str,
|
||||
code(
|
||||
[
|
||||
48,
|
||||
2,
|
||||
clampAndTruncate(color.r),
|
||||
clampAndTruncate(color.g),
|
||||
clampAndTruncate(color.b),
|
||||
],
|
||||
49
|
||||
)
|
||||
);
|
||||
}
|
||||
|
||||
// https://github.com/chalk/ansi-regex/blob/02fa893d619d3da85411acc8fd4e2eea0e95a9d9/index.js
|
||||
const ANSI_PATTERN = new RegExp(
|
||||
[
|
||||
'[\\u001B\\u009B][[\\]()#;?]*(?:(?:(?:(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]+)*|[a-zA-Z\\d]+(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]*)*)?\\u0007)',
|
||||
'(?:(?:\\d{1,4}(?:;\\d{0,4})*)?[\\dA-PR-TZcf-nq-uy=><~]))',
|
||||
].join('|'),
|
||||
'g'
|
||||
);
|
||||
|
||||
export function stripColor(string: string): string {
|
||||
return string.replace(ANSI_PATTERN, '');
|
||||
}
|
@ -1,12 +1,23 @@
|
||||
{
|
||||
"include": [
|
||||
"index.mjs"
|
||||
],
|
||||
"$schema": "https://json.schemastore.org/tsconfig",
|
||||
"compilerOptions": {
|
||||
"module": "Node16",
|
||||
"outDir": "dist",
|
||||
"allowJs": true,
|
||||
"composite": false,
|
||||
"declaration": true,
|
||||
"emitDeclarationOnly": true
|
||||
}
|
||||
"declarationMap": true,
|
||||
"esModuleInterop": true,
|
||||
"forceConsistentCasingInFileNames": true,
|
||||
"inlineSources": false,
|
||||
"isolatedModules": true,
|
||||
"moduleResolution": "node",
|
||||
"noUnusedLocals": false,
|
||||
"noUnusedParameters": false,
|
||||
"preserveWatchOutput": true,
|
||||
"skipLibCheck": true,
|
||||
"strict": true,
|
||||
"importsNotUsedAsValues": "error",
|
||||
"lib": ["ESNext"],
|
||||
"target": "es2020",
|
||||
"module": "es2022"
|
||||
},
|
||||
"exclude": ["node_modules"]
|
||||
}
|
||||
|
Loading…
x
Reference in New Issue
Block a user