mirror of
https://github.com/sqlite/sqlite.git
synced 2024-11-22 03:40:55 +01:00
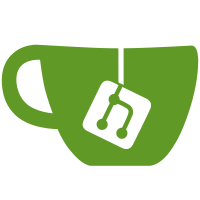
have both a text and a numeric type, make sure the numeric type does not confuse the answer. This is a deeper fix to the problem observed by [forum:/forumpost/3776b48e71|forum pose 3776b48e71]. The problem bisects to [25f2246be404f38b] on 2014-08-24, prior to version 3.8.7. FossilOrigin-Name: 709841f88c77276f09701bf38e25503c64b3a0afbe2fbf878136db12f31cbe21
91 lines
2.5 KiB
C
91 lines
2.5 KiB
C
/*
|
|
** 2020-01-08
|
|
**
|
|
** The author disclaims copyright to this source code. In place of
|
|
** a legal notice, here is a blessing:
|
|
**
|
|
** May you do good and not evil.
|
|
** May you find forgiveness for yourself and forgive others.
|
|
** May you share freely, never taking more than you give.
|
|
**
|
|
******************************************************************************
|
|
**
|
|
** This SQLite extension implements a noop() function used for testing.
|
|
**
|
|
** Variants:
|
|
**
|
|
** noop(X) The default. Deterministic.
|
|
** noop_i(X) Deterministic and innocuous.
|
|
** noop_do(X) Deterministic and direct-only.
|
|
** noop_nd(X) Non-deterministic.
|
|
*/
|
|
#include "sqlite3ext.h"
|
|
SQLITE_EXTENSION_INIT1
|
|
#include <assert.h>
|
|
#include <string.h>
|
|
|
|
/*
|
|
** Implementation of the noop() function.
|
|
**
|
|
** The function returns its argument, unchanged.
|
|
*/
|
|
static void noopfunc(
|
|
sqlite3_context *context,
|
|
int argc,
|
|
sqlite3_value **argv
|
|
){
|
|
assert( argc==1 );
|
|
sqlite3_result_value(context, argv[0]);
|
|
}
|
|
|
|
/*
|
|
** Implementation of the multitype_text() function.
|
|
**
|
|
** The function returns its argument. The result will always have a
|
|
** TEXT value. But if the original input is numeric, it will also
|
|
** have that numeric value.
|
|
*/
|
|
static void multitypeTextFunc(
|
|
sqlite3_context *context,
|
|
int argc,
|
|
sqlite3_value **argv
|
|
){
|
|
assert( argc==1 );
|
|
(void)argc;
|
|
(void)sqlite3_value_text(argv[0]);
|
|
sqlite3_result_value(context, argv[0]);
|
|
}
|
|
|
|
#ifdef _WIN32
|
|
__declspec(dllexport)
|
|
#endif
|
|
int sqlite3_noop_init(
|
|
sqlite3 *db,
|
|
char **pzErrMsg,
|
|
const sqlite3_api_routines *pApi
|
|
){
|
|
int rc = SQLITE_OK;
|
|
SQLITE_EXTENSION_INIT2(pApi);
|
|
(void)pzErrMsg; /* Unused parameter */
|
|
rc = sqlite3_create_function(db, "noop", 1,
|
|
SQLITE_UTF8 | SQLITE_DETERMINISTIC,
|
|
0, noopfunc, 0, 0);
|
|
if( rc ) return rc;
|
|
rc = sqlite3_create_function(db, "noop_i", 1,
|
|
SQLITE_UTF8 | SQLITE_DETERMINISTIC | SQLITE_INNOCUOUS,
|
|
0, noopfunc, 0, 0);
|
|
if( rc ) return rc;
|
|
rc = sqlite3_create_function(db, "noop_do", 1,
|
|
SQLITE_UTF8 | SQLITE_DETERMINISTIC | SQLITE_DIRECTONLY,
|
|
0, noopfunc, 0, 0);
|
|
if( rc ) return rc;
|
|
rc = sqlite3_create_function(db, "noop_nd", 1,
|
|
SQLITE_UTF8,
|
|
0, noopfunc, 0, 0);
|
|
if( rc ) return rc;
|
|
rc = sqlite3_create_function(db, "multitype_text", 1,
|
|
SQLITE_UTF8,
|
|
0, multitypeTextFunc, 0, 0);
|
|
return rc;
|
|
}
|