# Hono Hono[炎] - _**means flame🔥 in Japanese**_ - is small, simple, and ultrafast web framework for a Service Workers API based serverless such as Cloudflare Workers and Fastly Compute@Edge. ```js import { Hono } from 'hono' const app = new Hono() app.get('/', (c) => c.text('Hono!!')) app.fire() ``` ## Features - **Ultra fast** - the router is implemented with Trie-Tree structure. Not use loops. - **Zero dependencies** - using only Web standard API. - **Middleware** - builtin middleware, and you can make your own middleware. - **Optimized** - for Cloudflare Workers. ## Benchmark **Hono is fastest** compared to other routers for Cloudflare Workers. ```plain hono x 779,197 ops/sec ±6.55% (78 runs sampled) itty-router x 161,813 ops/sec ±3.87% (87 runs sampled) sunder x 334,096 ops/sec ±1.33% (93 runs sampled) worktop x 212,661 ops/sec ±4.40% (81 runs sampled) Fastest is hono ✨ Done in 58.29s. ``` ## Hono in 1 minute Below is a demonstration to create an application of Cloudflare Workers with Hono. 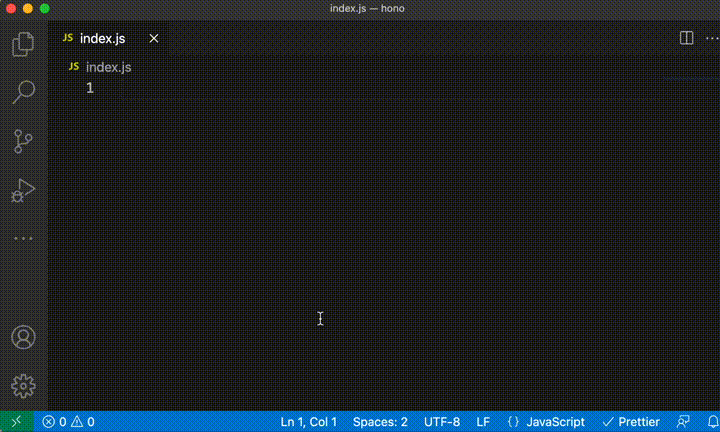 Now, the named path parameter has types. 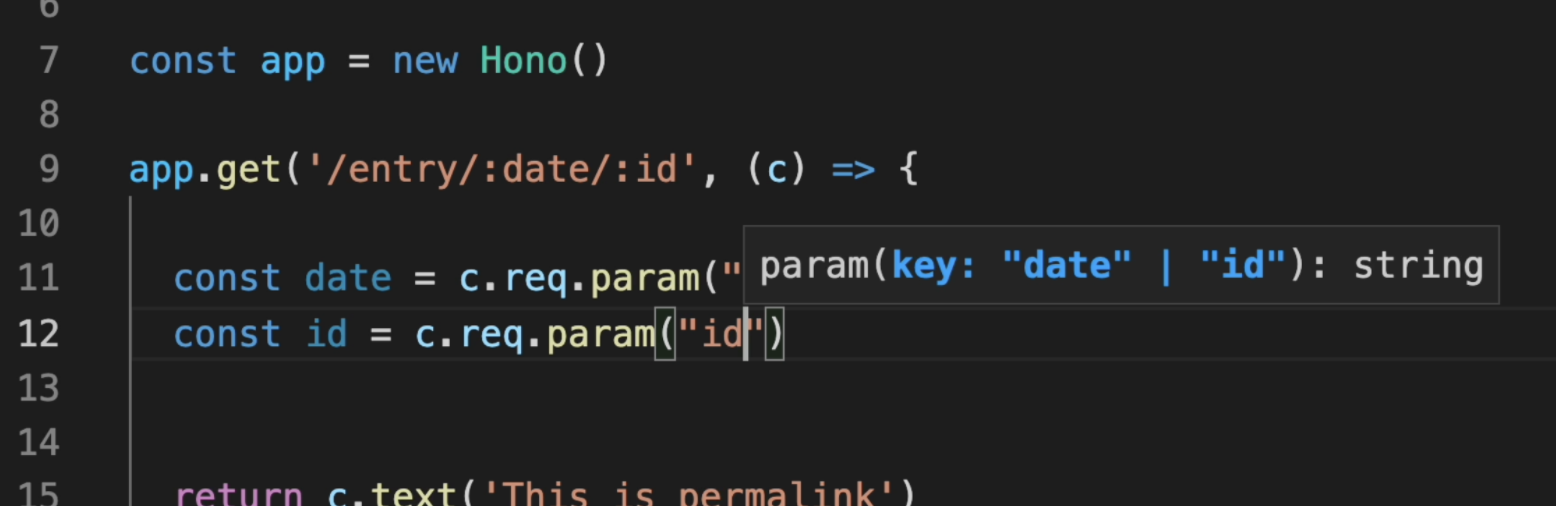 ## Install You can install Hono from npm registry: ```sh yarn add hono ``` or ```sh npm install hono ``` ## Methods An instance of `Hono` has these methods: - app.**HTTP_METHOD**(path, handler) - app.**all**(path, handler) - app.**route**(path) - app.**use**(path, middleware) - app.**fire**() - app.**fetch**(request, env, event) ## Routing ### Basic `app.HTTP_METHOD` ```js // HTTP Methods app.get('/', (c) => c.text('GET /')) app.post('/', (c) => c.text('POST /')) // Wildcard app.get('/wild/*/card', (c) => { return c.text('GET /wild/*/card') }) ``` `app.all` ```js // Any HTTP methods app.all('/hello', (c) => c.text('Any Method /hello')) ``` ### Named Parameter ```js app.get('/user/:name', (c) => { const name = c.req.param('name') ... }) ``` ### Regexp ```js app.get('/post/:date{[0-9]+}/:title{[a-z]+}', (c) => { const date = c.req.param('date') const title = c.req.param('title') ... ``` ### Nested route ```js const book = app.route('/book') book.get('/', (c) => c.text('List Books')) // => GET /book book.get('/:id', (c) => { // => GET /book/:id const id = c.req.param('id') return c.text('Get Book: ' + id) }) book.post('/', (c) => c.text('Create Book')) // => POST /book ``` ### no strict If `strict` is set `false`, `/hello`and`/hello/` are treated the same: ```js const app = new Hono({ strict: false }) app.get('/hello', (c) => c.text('/hello or /hello/')) ``` Default is `true`. ### async/await ```js app.get('/fetch-url', async (c) => { const response = await fetch('https://example.com/') return c.text(`Status is ${response.status}`) }) ``` ## Middleware ### Builtin Middleware ```js import { Hono } from 'hono' import { poweredBy } from 'hono/powered-by' import { logger } from 'hono/logger' import { basicAuth } from 'hono/basicAuth' const app = new Hono() app.use('*', poweredBy()) app.use('*', logger()) app.use('/auth/*', basicAuth({ username: 'hono', password: 'acoolproject' })) ``` Available builtin middleware are listed on [src/middleware](https://github.com/yusukebe/hono/tree/master/src/middleware). ### Custom Middleware You can write your own middleware: ```js // Custom logger app.use('*', async (c, next) => { console.log(`[${c.req.method}] ${c.req.url}`) await next() }) // Add a custom header app.use('/message/*', async (c, next) => { await next() await c.header('x-message', 'This is middleware!') }) app.get('/message/hello', (c) => c.text('Hello Middleware!')) ``` ## Context To handle Request and Reponse, you can use Context object: ### c.req ```js // Get Request object app.get('/hello', (c) => { const userAgent = c.req.headers.get('User-Agent') ... }) // Shortcut to get a header value app.get('/shortcut', (c) => { const userAgent = c.req.header('User-Agent') ... }) // Query params app.get('/search', (c) => { const query = c.req.query('q') ... }) // Captured params app.get('/entry/:id', (c) => { const id = c.req.param('id') ... }) ``` ### Shortcuts for Response ```js app.get('/welcome', (c) => { c.header('X-Message', 'Hello!') c.header('Content-Type', 'text/plain') c.status(201) return c.body('Thank you for comming') /* Same as: return new Response('Thank you for comming', { status: 201, statusText: 'Created', headers: { 'X-Message': 'Hello', 'Content-Type': 'text/plain', 'Content-Length: '22' } }) */ }) ``` ### c.text() Render text as `Content-Type:text/plain`: ```js app.get('/say', (c) => { return c.text('Hello!') }) ``` ### c.json() Render JSON as `Content-Type:application/json`: ```js app.get('/api', (c) => { return c.json({ message: 'Hello!' }) }) ``` ### c.html() Render HTML as `Content-Type:text/html`: ```js app.get('/', (c) => { return c.html('